In this post you’ll learn how to create regular expression by yourself and how to start practicing while working with Visual Studio. Considering you’re working on a large codebase and regularly you need to search for method names, Event names or just some code lines or anything that you want to look up in the code base. Usually what developers do use is “Find in Files” or “Find All” with given terms. But what if you don’t know the exact name of the code line you want to search. What will you do? Well, you can definitely think of a patterns that may help you find your target line of code.
I have this EntityFramework 6 code base forked from Git repository. This is a pretty large code base to look into something. So For e.g. I want to look for all classes used in validation. I’ll start my search with following in mind:
- Assuming they’re named properly with the prefix, suffix or in between the name of a classes.
- The classes names may contain variations like “Validator”, “Validation”, Validatable” etc.
Either I can search for all these terms individually and collect results manually, Or I can use pattern matching and find all result in one go. So I’m going to open the “Find in files” window(SHIFT + CTRL + F) and start writing a Regular expression for it:

I’m using the expression .* class \w+Valida\w+ (I’ll explain next what it means) to find a patterns based on my assumptions above. After clicking on “Find All” I have following results:

As you can see all my desired classes are listed in the results “Viola!!”.
Instead of searching for individual keywords I have my result in single search. I saved time and I have my regular expression which might not be perfect and it did the job.
Explanation of Regex used aboveLet break down this and see what individual things means:
. – Represents anything(character-non character, symbols, digits etc.) in a string.
* – Represents 0 or more occurrences.
class – Represents a string literal and it will be an exact match.
\w – Represents any alphanumeric and including _ (underscore).
+ – Represents 1 or more occurrences.
Valida - Represents a string literal and it will be an exact match
\w+ – As explained above.
Well, this was easy or is it not?
Another simplest case I can mention here which every developer might have faced is managing Spaces in Code, in Text or in Strings at run time. A simple way to remove additional spaces using regular expression is, (I’m using C# Regex library for this demo):
Regex.Replace(“ Some Text with un even spaces”, “(\s+)”, “(\s)”).Trim();
Output -
“Some Text with un even spaces”
Let’s go back a little and start with basics and then go step by step:
Step 1 – If you are completely new to the Regular Expressions then you can start with a free practice website which provide insights and great details of each exercise https://regexone.com
Explanation of symbols are provided in the right side column. But I’m also going to use the screenshot from the website:
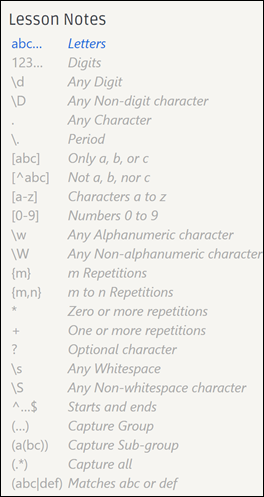
This will give you pretty good explanation of each special character and symbols used in Regualar Expressions.
After memorizing each and every of these notation you can start taking the exercises one by one. And in hardly 1-2 hours of practice you will be able to write you first Regular Expression without any help.
Another source to learn and test my regex I prefer to https://regex101.com
This site provide better insights of your regular expression on the fly. But I would say once you are on the next level and have confident go the website and challenge your Regex with various tests and insights including optimization of your Regular Expression.
Step 2 – Now that you have learned to write the basic regular expression. It’s time to test your skills in visual studio. As I stated an example above you can start using Regular expression as search option instead of searching for “Contains” search in visual studio. And at this step you are already stepped into the Advanced user of visual studio.
Step 3 – Creating complex expression and getting help right from visual studio. If you don’t know then Let me show you that visual studio have it’s built-in helper utility to create regular expression right from visual studio.
Open the “Find in Files” dialog window, Select “Regular Expression” Like a boss.

Then click on button next to the “Find What:” input box. And you’ll see the hidden treasure:
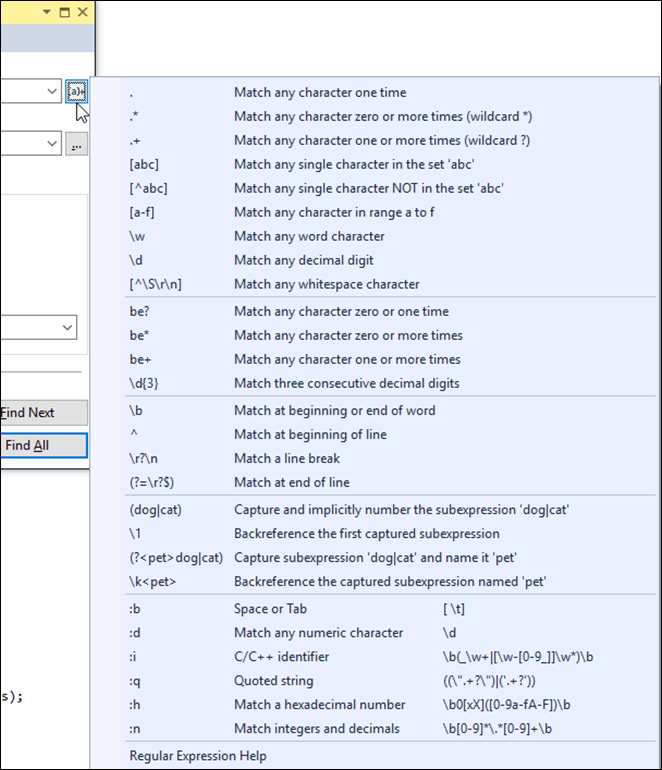
These are the tricks at your finger tips. Now you can start writing and testing your regex using “Quick Find” as well. It shows you the live match results by highlighting the matching result with in the Editor window.
So for e.g. I wan to search anything that start with Collection then here’s my quick tip, Just go to “Quick Find’'” (CTRL + F) and write your regex:


With the combination of search and replace you can easily refactor your code without repeating steps and saving a lot of time for other stuff.
Testing your Random Regular Expression:
You can again use the Visual studio editor to test your regex which you might be going to use in your code. How? Simply create a text file with your sample Text data. E.g. you want to capture cell phone from a Text,
/* == Matching cell phone numbers == */
3473459090
12123232111
ABdfasdfasdlf
Another test string
1106 is a zip code
but this is a cell phone number +1 121 212 2241
121 212 2241
Now open the quick find box and put your Regular Expression in there and see what is matching and what is not.
For e.g. I prepared a Regex to find the phone numbers as 1?[\s-]?\(?(\d{3})\)?[\s-]?\d{3}[\s-]?\d{4}
Let’s see how my results are looking:

Now instead of going to some online website to test your regex you can directly give a dry run with in Visual studio. Hope you have had fun reading and learning one of the essential skills of quick smart developer which can be you. Keep practicing!!